How to make API calls from Custom Code actions inside HubSpot Workflows
by Richard on Jun 10, 2024
Learn how to leverage external API calls from custom code actions within HubSpot Workflows to enhance automation and data processing.
Understanding Custom Code Actions in Hubspot Workflows
Custom code actions in HubSpot Workflows allow you to extend the functionality of your workflows by adding your own code snippets. These snippets can be written in a variety of programming languages, such as JavaScript or Python, and can interact with external resources like APIs. Custom code actions give you the flexibility to perform complex operations and integrate with other systems.
By using custom code actions, you can go beyond the built-in actions provided by HubSpot and create custom logic that suits your specific needs. This opens up a world of possibilities for automating tasks and enhancing data processing within your HubSpot workflows.
Exploring Use Cases for Custom Code Actions
Custom code actions can be used in a wide range of scenarios to enhance your HubSpot workflows. Some common use cases include:
- Fetching data from external APIs and integrating it into your HubSpot account
- Performing complex calculations and data transformations
- Sending data to external systems or services
- Implementing custom business rules and validations
By leveraging custom code actions, you can tailor your workflows to meet your specific business requirements and streamline your operations.
Adding a Custom Code Action to a HubSpot Workflow
Before we start coding, we need a place for it. To add a custom code action you have to go the main workflow menu where all the actions live, search for "custom code" and click on it.
Now you can start configuring the action and you can add all the properties you need to include in the code editor below. For this guide, we will add the firstname property and leave the default label.
Making External API Calls from Custom Code Actions
One of the powerful capabilities of custom code actions in HubSpot Workflows is the ability to make external API calls. This allows you to retrieve data from external sources and incorporate it into your workflows.
To make an external API call from a custom code action, you can use libraries or native language features to send HTTP requests to the API endpoint. You can then process the response data and use it in your workflow logic.
In the next section, we will explore an example of making an API call using a public API called genderize.io to predict the gender of a person based on their name.
Implementing API Call to Predict Gender
In this example, we will demonstrate how to make an API call to the genderize.io API to predict the gender of a person based on their name.
First, in the code editor we add the axios library:
const axios = require('axios');
Then, inside exports.main, we create our variable for the first name. Remember you have to add this property in the "Property to include in code" section.
const firstname = event.inputFields['firstname'];
Now we will create the function that will send the request. In this code, we use the axios library to send a GET request to the genderize.io API with the name as a query parameter. The API will then return a response containing the predicted gender.
async function predictGender(name) {
try {
const response = await axios.get(`https://api.genderize.io/?name=${name}`);
return response.data.gender;
} catch (error) {
console.error(error);
throw new Error('Failed to predict gender.');
}
}
We will then define our gender variable which will store the API's response by calling our predictGender function:
let gender;
try {
gender = await predictGender(firstname);
} catch (error) {
gender = 'unknown'; // Default value if there is an error
}
Now we have to write our callback and pass along the variables:
callback({
outputFields: {
firstname: firstname,
gender: gender
}
});
And that's it! Here is the final code snippet:
const axios = require('axios');
exports.main = async (event, callback) => {
// Create the firstname variable
const firstname = event.inputFields['firstname'];
// Function to predict gender based on name
async function predictGender(name) {
try {
const response = await axios.get(`https://api.genderize.io/?name=${name}`);
return response.data.gender;
} catch (error) {
console.error(error);
throw new Error('Failed to predict gender.');
}
}
// Calling the predictGender function and response handling
let gender;
try {
gender = await predictGender(firstname);
} catch (error) {
gender = 'unknown';
}
// Using the callback function to handle the output fields
callback({
outputFields: {
firstname: firstname,
gender: gender
}
});
}
Once we have our code, we need to add the gender variable as an output so we can use it later in other actions. In the next section we will explain how to do this.
Setting HubSpot Property Value with API Response
Once you have obtained the gender prediction from the API call, you can use the response data to set the value of a HubSpot property.
To achieve this, you can either use the HubSpot API to update the desired property of a contact with the predicted gender or you can use the default "Set a property value" HubSpot action. For simplicity, we will use the default action to set a property value with the custom code output we created.
First let's add the output as a string and call it gender. Note that the name of this output should be the same as the one you defined in your callback:
Save your custom code action. Now we can add the "Set property value" action to the workflow:
We will now choose the Contact object and the Gender property, which will store our gender. In the side menu that opens we have to choose the data that we want to insert, we search for "Action outputs" (these are the outputs from our custom code) and then click on gender output:
By setting the HubSpot property value with the custom code output, you can enrich your contact data and use it for segmentation, personalization, or any other purposes in your HubSpot workflows.
I hope this guide has provided you with a clear understanding of how to make external API calls using HubSpot's workflow custom code action. By leveraging this powerful feature, you can significantly enhance your workflows and automate more complex tasks with ease.
If you have any doubts or need further clarification, please feel free to leave a comment below – I'm here to help! And if you found this post helpful, don't hesitate to share it with your network. Your support is greatly appreciated!
Happy automating!
You May Also Like
These Related Stories
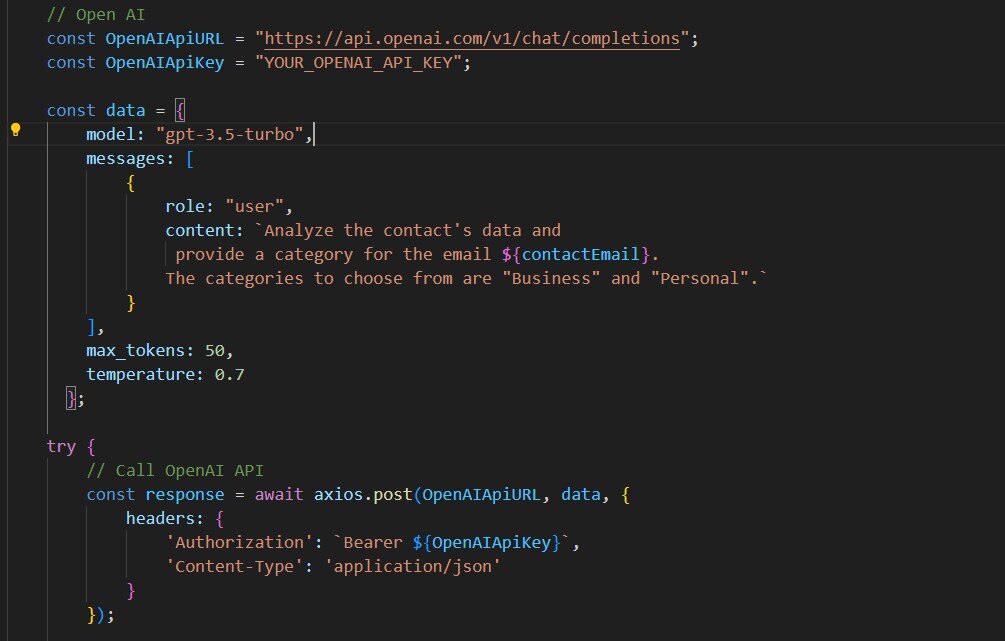
How to Use AI in HubSpot Workflows
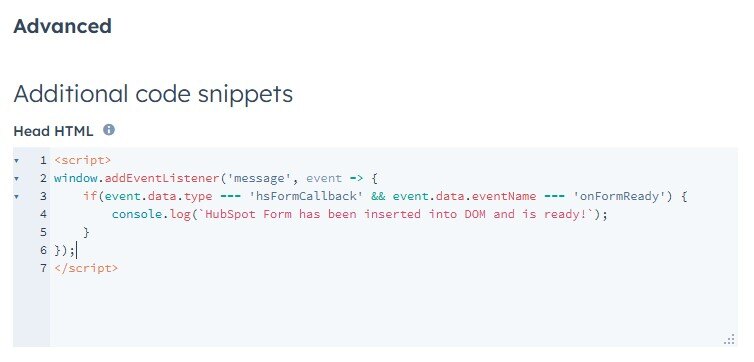
How to Track HubSpot Form Submission Events with Javascript
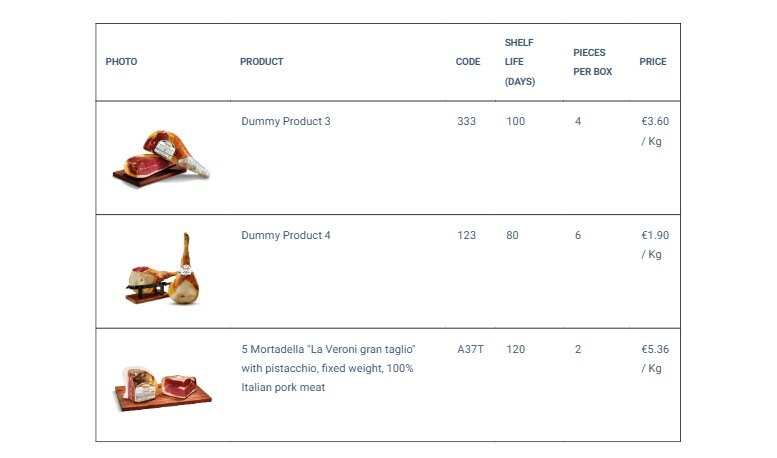
No Comments Yet
Let us know what you think